How to Create an Efficient Dockerfile for Python Projects (Step-by-Step Guide)
In this guide, you’ll learn how to create an efficient Dockerfile for Python, along with explanations for each step and optimization tips that help your app run smoothly in any environment.
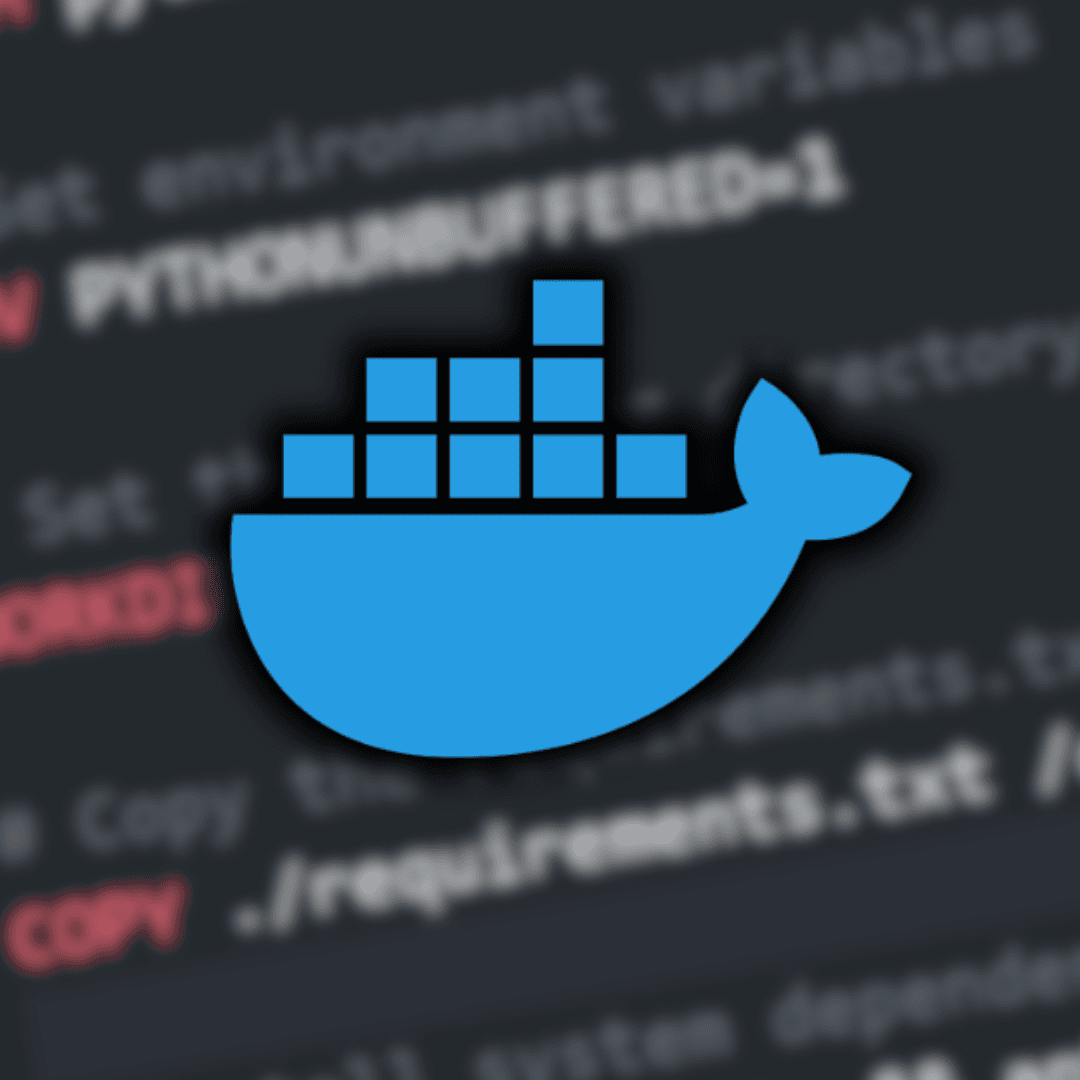
Introduction
If you're developing a Python application and want to containerize it using Docker, crafting a lean, efficient Dockerfile is essential. A well-optimized Dockerfile ensures faster builds, smaller image sizes, and smoother deployments—key factors that also impact SEO, user experience, and CI/CD performance.
In this guide, you’ll learn how to create an efficient Dockerfile for Python, along with explanations for each step and optimization tips that help your app run smoothly in any environment.
Why Dockerize a Python App?
Before diving in, here’s why developers Dockerize Python apps:
- Environment consistency: No more "it works on my machine."
- Scalability: Easily deploy to cloud providers or orchestrators like Kubernetes.
- CI/CD Integration: Works seamlessly in automation pipelines.
- Faster onboarding: Developers can start working without configuring environments.
Prerequisites
Before you begin, make sure you have:
- Python project (e.g., Flask, Django, FastAPI)
- requirements.txt file
- Docker installed locally
- Basic knowledge of Docker and Python
Step-by-Step: How to Create an Efficient Dockerfile for Python
🧱 1. Start with the Right Base Image
Choose a lightweight base image like python:3.X-slim . Avoid full images unless necessary.
dockerfile
FROM python:3.11-slim
✅ Why? Slim images are smaller and faster to pull, reducing build time and attack surface.
📁 2. Set the Working Directory
Define a working directory to keep things organized.
dockerfile
WORKDIR /app
📦 3. Install System Dependencies
Some Python packages may require system-level libraries.
dockerfile
RUN apt-get update && apt-get install -y \
build-essential \
libpq-dev \
&& rm -rf /var/lib/apt/lists/*
✅ Pro tip: Remove cache with rm -rf /var/lib/apt/lists/* to keep the image size minimal.
Also Read: How to Become a Top-Standard Developer in the IT Sector in 2025
📄 4. Copy Dependency Files Separately
Copy requirements.txt first to take advantage of Docker layer caching.
dockerfile
COPY requirements.txt .
🔧 5. Install Python Dependencies
Always use a virtual environment or install with --no-cache-dir :
dockerfile
RUN pip install --no-cache-dir -r requirements.txt
📦 6. Copy Your Application Code
Now copy the rest of your app into the image:
dockerfile
COPY . .
🚀 7. Set Environment Variables
Add environment variables for cleaner logging and security.
dockerfile
ENV PYTHONDONTWRITEBYTECODE=1
ENV PYTHONUNBUFFERED=1
✅ PYTHONDONTWRITEBYTECODE=1 prevents writing .pyc files.
✅ PYTHONUNBUFFERED=1 ensures logs are printed directly (useful for Docker logs).
🏁 8. Define the Default Command
Use a non-root user and specify how to run the app.
Example with FastAPI and Uvicorn:
dockerfile
CMD ["uvicorn", "main:app", "--host", "0.0.0.0", "--port", "8000"]
For Flask:
dockerfile
CMD ["python", "app.py"]
✅ Complete Efficient Dockerfile Example
dockerfile
FROM python:3.11-slim
# Set environment variables
ENV PYTHONDONTWRITEBYTECODE=1
ENV PYTHONUNBUFFERED=1
# Set working directory
WORKDIR /app
# Install dependencies
RUN apt-get update && apt-get install -y \
build-essential \
libpq-dev \
&& rm -rf /var/lib/apt/lists/*
# Install Python packages
COPY requirements.txt .
RUN pip install --no-cache-dir -r requirements.txt
# Copy app code
COPY . .
# Run the app (change based on your app entry point)
CMD ["python", "app.py"]
Bonus Tips to Optimize Further
✅ .dockerignore:
Use a .dockerignore file to skip unnecessary files and speed up builds:
__pycache__
*.pyc
*.pyo
.env
.git
✅ Multi-stage builds (for compiled apps):
Use multiple stages to compile in one stage and copy only the final output to the last stage.
✅ Security:
Avoid running as root. Create a user and use USER directive.
✅ Image Scanners:
Use tools like Docker Scout, Trivy, or Snyk to scan your image for vulnerabilities.
Conclusion
Creating an efficient Dockerfile for Python is more than just putting commands together—it's about optimizing for performance, maintainability, and security. By following the steps above, you can build lightweight, production-ready containers for your Python apps that are easier to deploy and scale.
You may also like
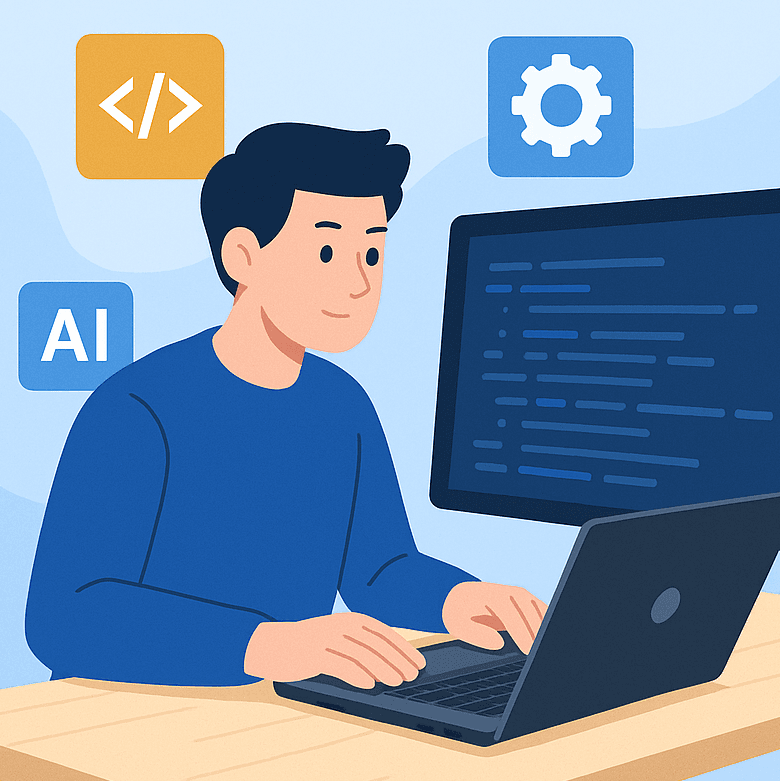
How to Become a Top-Standard Developer in the IT Sector in 2025
Summary
Read Full
open_in_newWith the rapid rise of AI, quantum computing, and remote-first technologies, being a standard developer is no longer enough. In 2025, IT professionals need to become "top-standard developers"—technologically adaptive, AI-savvy, and strategically aligned with market demands. This blog covers the key skills, tools, and trends to master in 2025 to become a top-performing developer in the global tech industry.
Post a comment
Comments
Most Popular
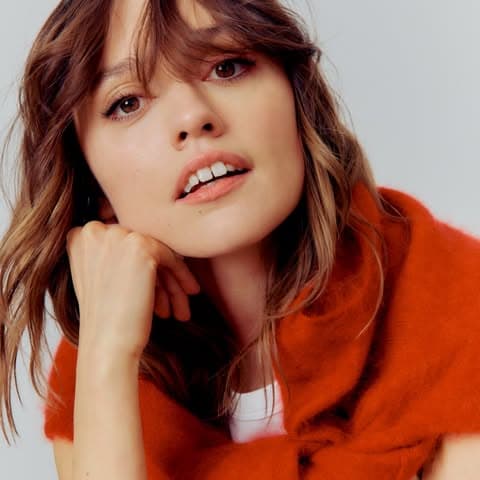
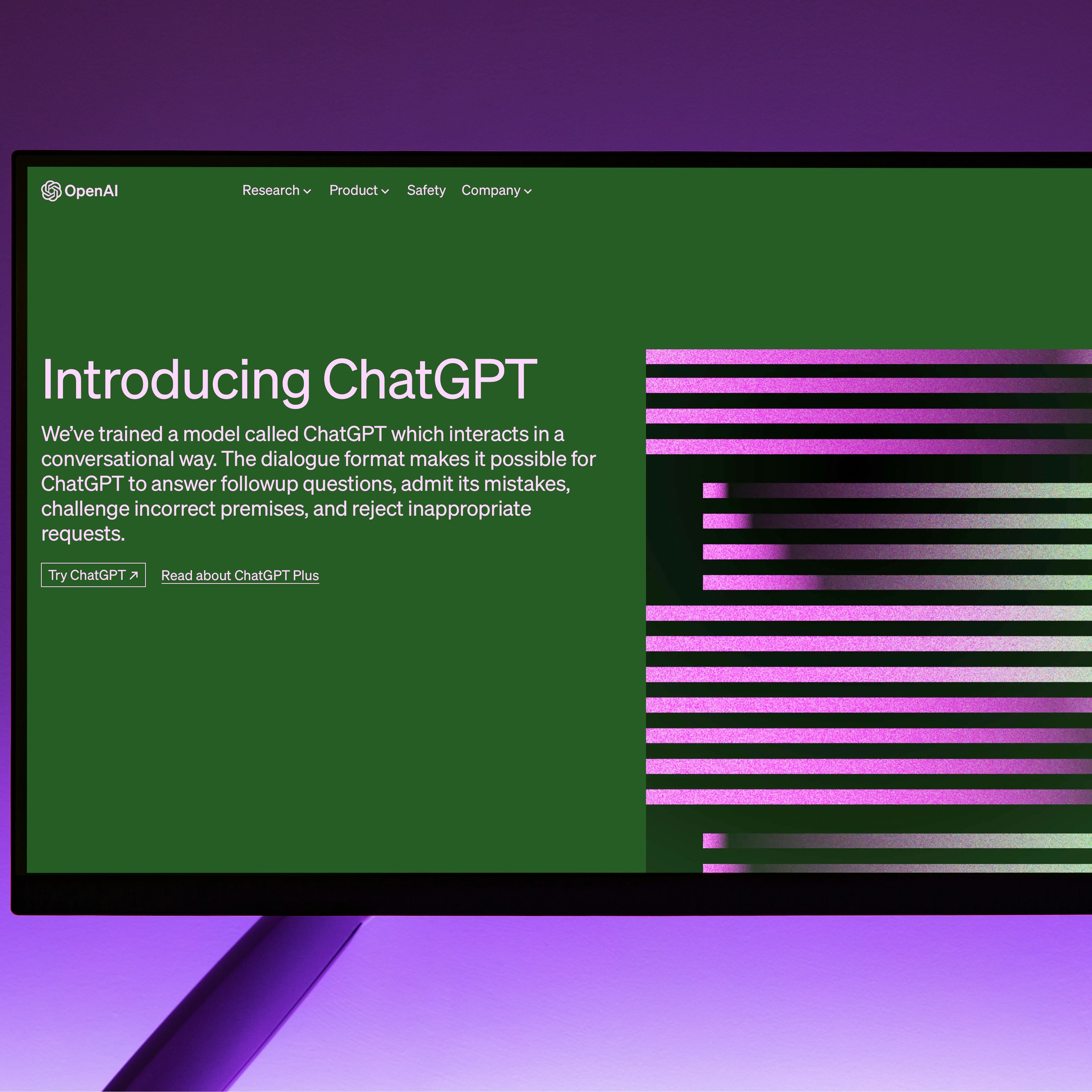
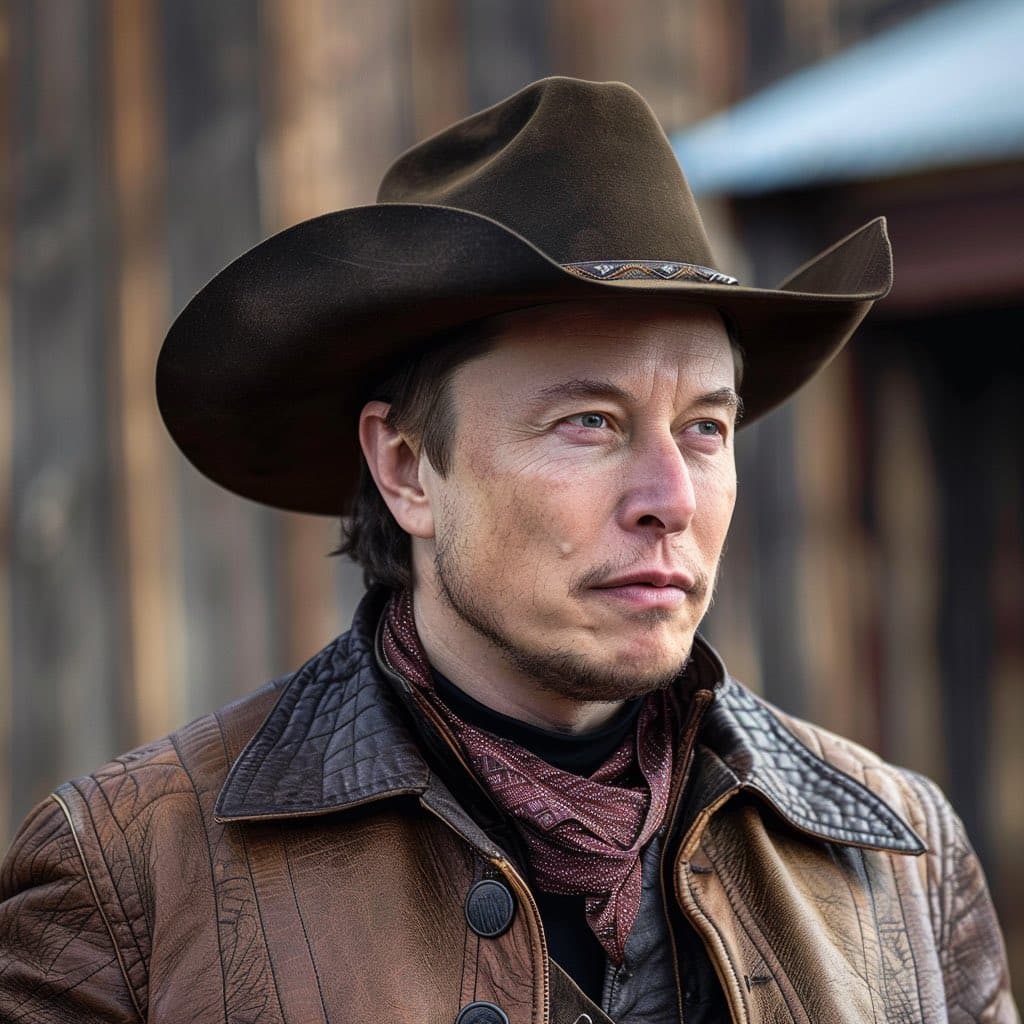
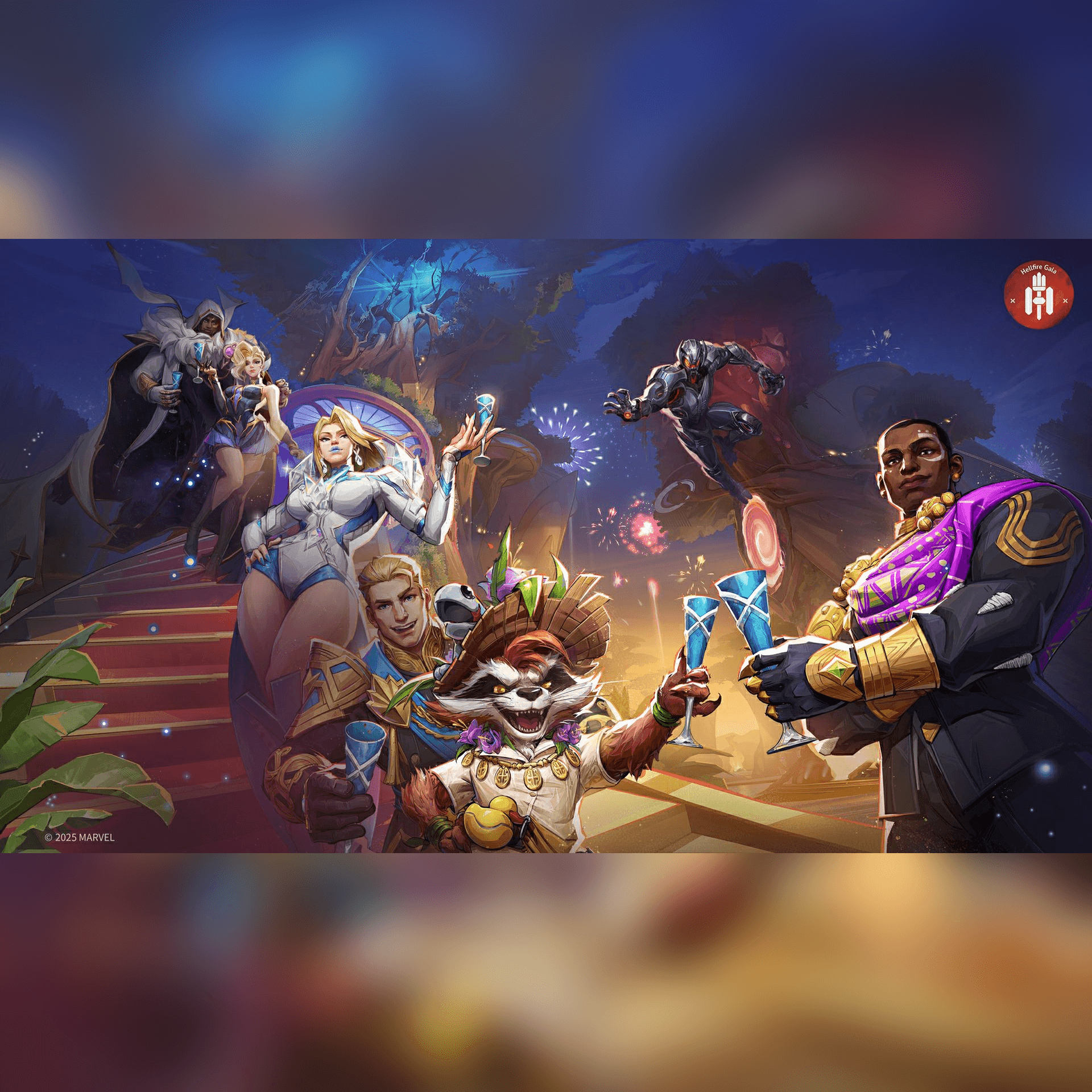
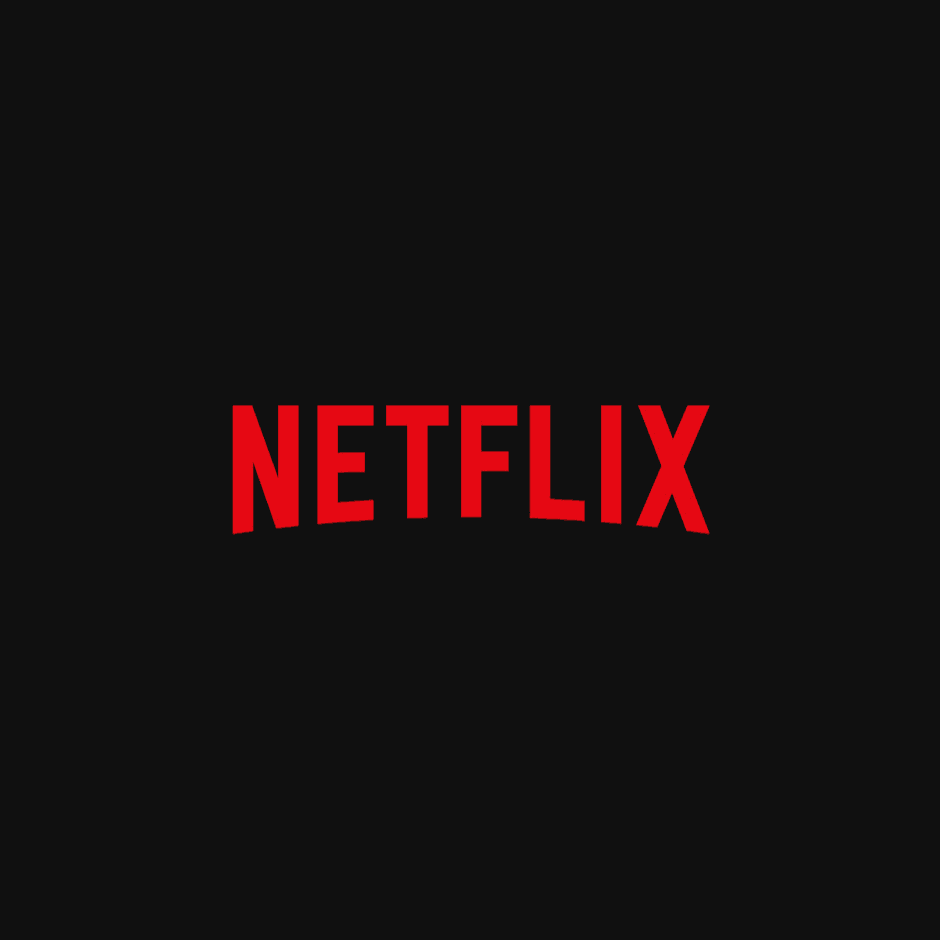
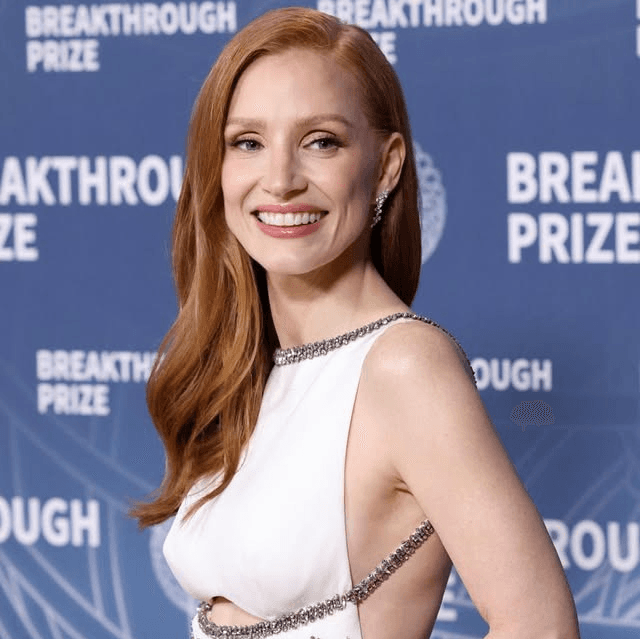
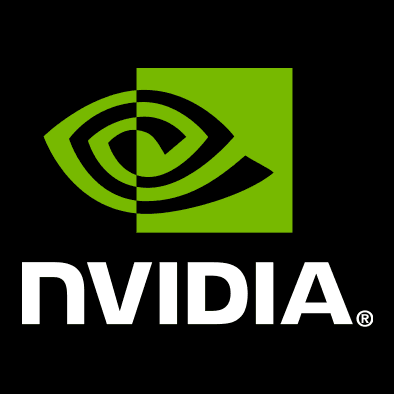
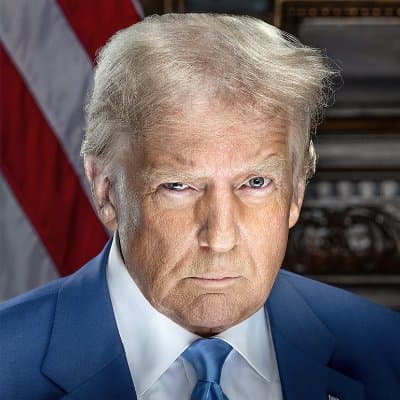
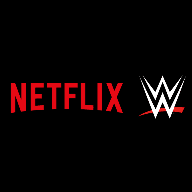
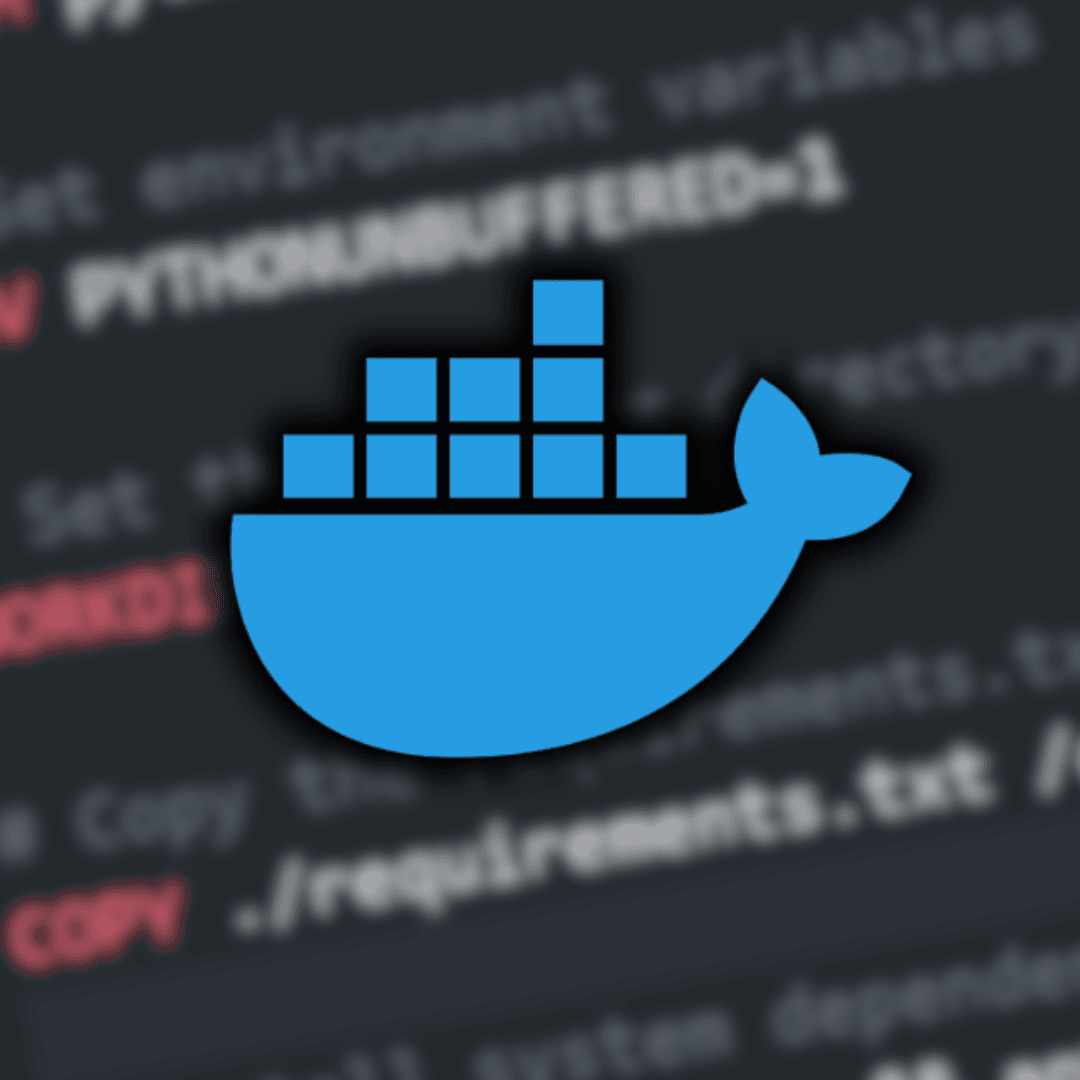